Warning most of the content may feel like AI because of the new AI revision system in the blogs
My Journey Learning CI/CD for Automated Deployment
One of my goals for this year was to learn about CI/CD (Continuous Integration and Continuous Deployment) to automate deployments and workflows. At the start of the year, I jumped into it, and it has been quite the experience! This post details my journey from manual deployments to automated pipelines.
Getting Started: The Lightbulb Moment
I knew CI/CD meant "automate stuff," but actually building a pipeline felt like assembling IKEA furniture blindfolded. Tutorials bombarded me with terms like artifacts, workflows, runners, and pipelines, while Jenkins' XML configs looked like hieroglyphics. The learning curve was steep, but the benefits were worth it.
What finally clicked for me was realizing CI/CD isn't about perfection - it's about incremental automation. I didn't need to automate my entire workflow overnight. Starting with small, repetitive tasks made the journey manageable.
Choosing Between Jenkins and GitHub Actions: A Newbie's Perspective
As I researched, I realized I needed to pick a tool to get started. The two major options that stood out to me were Jenkins and GitHub Actions. Here's what I learned about each:
Jenkins:
- Self-hosted and highly customizable
- Huge plugin ecosystem (over 1,500 plugins!)
- Steeper learning curve (XML configs made my eyes cross)
- Requires maintaining your own infrastructure
- Great for complex enterprise pipelines
GitHub Actions:
- Cloud-hosted and integrated with GitHub
- YAML-based configuration (still tricky, but more human-readable)
- Gentler learning curve with template workflows
- Free for public repositories (huge for personal projects)
- Perfect for smaller projects and GitHub-centric workflows
Since I was already living in GitHub for version control, GitHub Actions felt like slipping into comfortable shoes. The ability to prototype directly in my existing repos was a game-changer.
My First Steps with GitHub Actions: Baby Steps to Confidence
Learning GitHub Actions wasn't as hard as I initially thought, though it did take some patience. I began by automating small projects hosted on my VPS. The workflow syntax was intuitive, and the integration with GitHub made testing and debugging easier.
My automation roadmap looked like this:
- ๐งน Code quality checks: Linting and formatting validation
- ๐งช Test automation: Running unit tests on every push
- ๐๏ธ Build processes: Compiling assets automatically
- ๐ Deployment magic: SSH-ing into my VPS without lifting a finger
What surprised me most was how these small wins built momentum. Each successful automation made me eager to tackle the next challenge.
The "Hello World" of CI/CD: From Panic to Pride
I started with tiny tasks, like running tests or linting code. My first workflow file wasn't anything fancy it was basic, but it worked!
name: CI/CD Training Wheels
on: [push]
jobs:
quality-checks:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: '18'
- name: Install dependencies
run: npm install
- name: Run linting
run: npm run lint
The first time I saw those green checkmarks appear automatically after a push, I felt like I'd unlocked a superpower. No more remembering to run linters manually - the pipeline became my safety net.
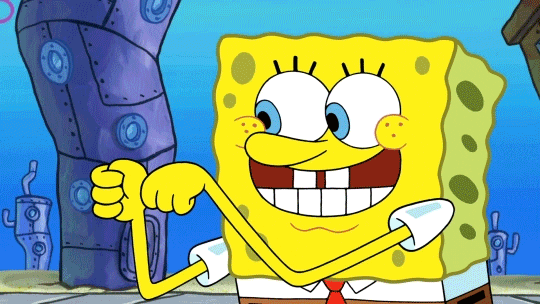
Mind-blown reaction GIF
From Manual SSH to Automated Bliss
Before CI/CD, my deployment process looked like this:
- Make code changes locally
- SSH into VPS
git pull
latest changesnpm install
if dependencies changed- Restart services
- Cross fingers and refresh browser
Now with GitHub Actions, I've automated 90% of this process. My current workflow:
- name: Deploy to VPS
uses: appleboy/ssh-action@master
with:
host: ${{ secrets.VPS_IP }}
username: ${{ secrets.VPS_USER }}
key: ${{ secrets.VPS_SSH_KEY }}
script: |
cd /var/www/my-app
git pull origin main
npm install --production
pm2 restart my-app
The magic happens automatically on every push to main. I've literally deployed fixes from my phone while commuting!
Jenkins: The Next Frontier
I've recently started dipping my toes into Jenkins. First impressions:
- Powerful but complex: The plugin ecosystem is amazing but overwhelming
- Flexibility comes at a cost: More control means more configuration
- Steep learning curve: Groovy syntax vs. YAML took some adjustment
My first Jenkins pipeline looks something like this:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'npm install'
}
}
stage('Test') {
steps {
sh 'npm test'
}
}
}
}
It's early days, but I'm excited to explore Jenkins' enterprise capabilities while still using GitHub Actions for personal projects.
Why Every Developer Should Try CI/CD
If you're a developer and haven't tried CI/CD yet, I highly recommend starting small:
- Automate your linters
- Set up basic tests
- Create a simple build process
- Gradually add deployment steps
The payoff is immense: fewer "it works on my machine" moments, consistent deployments, and more time for actual coding. Plus, there's something magical about watching your pipeline do the work for you! ๐ฉโจ